The loop control variable is initialized after entering the loop – Loop control variable initialization after entering the loop is a programming technique that offers unique advantages and considerations. This approach, where the loop control variable is set after the loop’s commencement, provides a distinct perspective on loop execution and performance optimization.
By delving into the concept of loop control variable initialization after loop entry, this discourse explores its significance, examines its impact on loop behavior, analyzes performance implications, and establishes best practices for effective implementation.
Loop Control Variable Initialization
Initializing a loop control variable is a crucial step in loop programming. It involves setting the initial value of the variable that controls the loop’s execution. Proper initialization ensures that the loop executes as intended, preventing potential errors and unexpected behavior.
The primary purpose of initializing the loop control variable is to establish a starting point for the loop’s iteration. Without initialization, the variable may contain an undefined value, leading to unpredictable results. Initialization also allows for greater control over the loop’s behavior, enabling programmers to specify the range and direction of the iteration.
In various programming languages, the syntax for initializing a loop control variable differs. For instance, in C++, the initialization is typically done within the loop’s header, as in:
int i = 0;for (i; i< 10; i++) // loop body
In Python, the initialization can be done before the loop:
i = 0for i in range(10): # loop body
Loop Control Variable Initialization Placement
The placement of the loop control variable initialization can significantly impact the loop’s behavior. Initializing the variable after entering the loop offers certain advantages and disadvantages compared to initializing before the loop.
One advantage of initializing after entering the loop is that it allows for more flexibility. It enables programmers to dynamically adjust the loop’s range or direction based on conditions encountered within the loop. For instance, the following C++ code initializes the loop control variable after entering the loop:
int i = 0;while (true) if (condition) i = 10; break; // loop body i++;
In this example, the loop continues indefinitely until the condition is met, at which point the loop control variable is initialized to 10 and the loop breaks. This approach provides greater control over the loop’s behavior.
However, initializing the loop control variable after entering the loop can also lead to potential drawbacks. If the initialization is not done properly, it can result in infinite loops or incorrect loop execution. Additionally, it can make the code less readable and maintainable.
Best practices dictate that the loop control variable should be initialized before entering the loop whenever possible. This ensures that the loop’s range and direction are clearly defined from the outset, making the code more predictable and easier to debug.
Impact on Loop Behavior
Initializing the loop control variable after entering the loop can have a significant impact on the loop’s behavior. Proper initialization ensures that the loop iterates as intended, while improper initialization can lead to unexpected results.
One potential consequence of not initializing the loop control variable properly is that the loop may enter an infinite loop. This occurs when the loop condition is always true and the loop control variable is never updated. For example, the following C++ code demonstrates an infinite loop due to improper initialization:
int i;while (i< 10) // loop body
In this example, the loop control variable i is not initialized before entering the loop. As a result, the loop will continue indefinitely because the condition i< 10 will always be true.
Another potential consequence of improper loop control variable initialization is that the loop may not execute at all. This can occur when the loop condition is initially false and the loop control variable is never updated. For instance, the following Python code demonstrates a loop that will not execute:
i = 10while i< 10: # loop body
In this example, the loop control variable i is initialized to 10 before entering the loop. Since the condition i< 10 is initially false, the loop will not execute at all.
Implications for Loop Performance, The loop control variable is initialized after entering the loop
The placement of the loop control variable initialization can have implications for the performance of the loop. In general, initializing the variable before entering the loop is more efficient than initializing it after entering the loop.
When the loop control variable is initialized before the loop, the compiler can optimize the loop’s execution. For instance, the compiler can determine the exact number of iterations of the loop and allocate memory accordingly. This can lead to improved performance, especially for loops with a large number of iterations.
On the other hand, initializing the loop control variable after entering the loop can result in decreased performance. This is because the compiler cannot optimize the loop’s execution as effectively. The compiler may not know the exact number of iterations of the loop, which can lead to less efficient memory allocation and slower execution.
To optimize loop performance, it is recommended to initialize the loop control variable before entering the loop whenever possible. This allows the compiler to optimize the loop’s execution and improve performance.
Key Questions Answered: The Loop Control Variable Is Initialized After Entering The Loop
What are the advantages of initializing the loop control variable after entering the loop?
Initializing the loop control variable after entering the loop allows for greater flexibility in loop construction, enables dynamic loop range determination, and potentially improves performance by avoiding unnecessary iterations.
What are the potential drawbacks of initializing the loop control variable after entering the loop?
Initializing the loop control variable after entering the loop can introduce potential errors if the initialization logic is incorrect, may result in infinite loops if the initialization condition is not met, and can impact code readability if not implemented carefully.
When is it appropriate to initialize the loop control variable after entering the loop?
Initializing the loop control variable after entering the loop is suitable when the loop range is not known in advance, when the loop needs to be executed conditionally, or when optimizing loop performance is a priority.
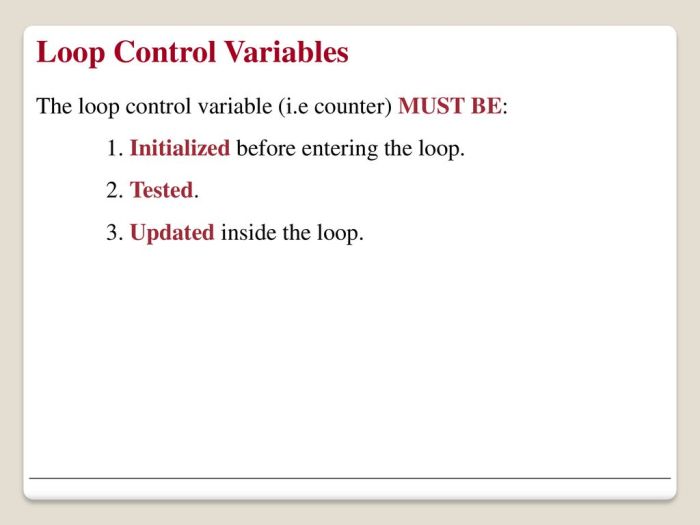
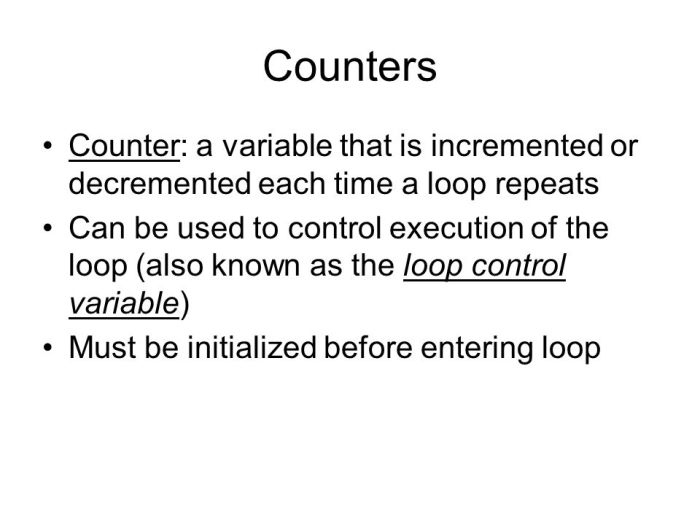
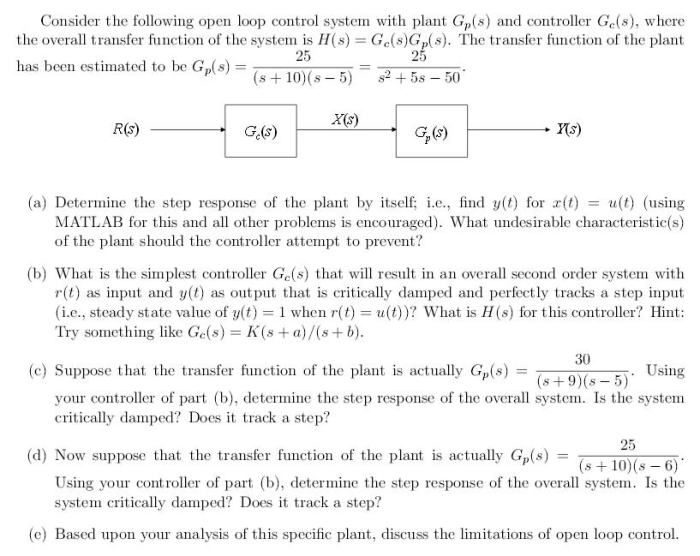